Chrome Extension: A shortcut for Meet's Picture-in-Picture mode
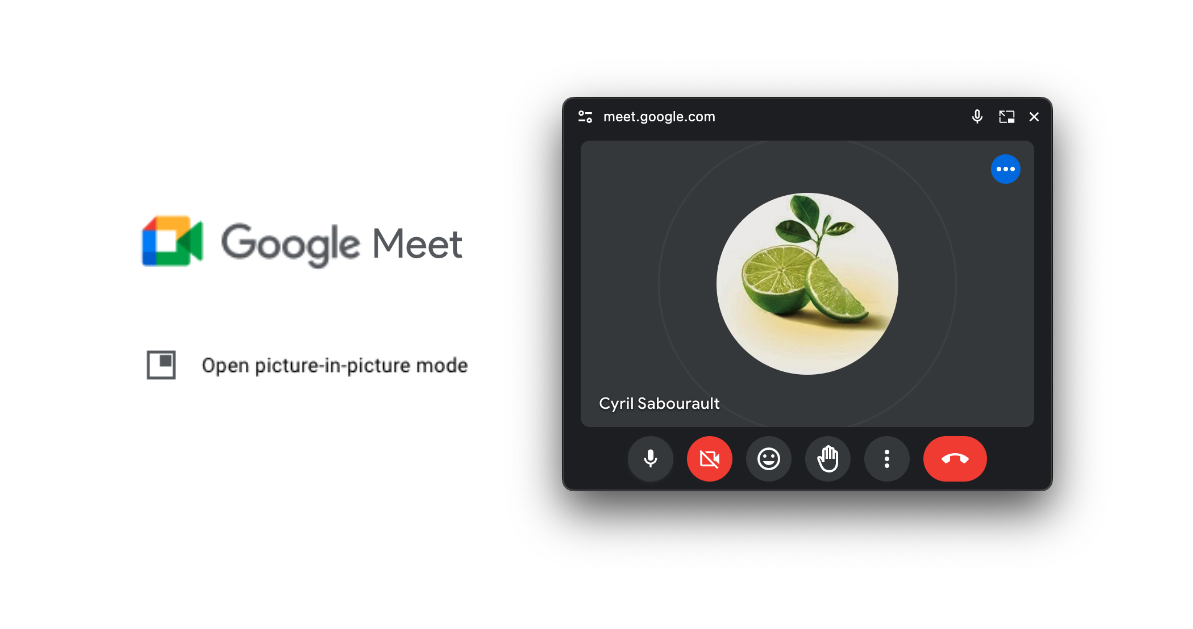
Chrome Extension: A shortcut for Meet’s Picture-in-Picture mode
The idea 💡
Having a keyboard shortcut to open the Picture-in-Picture mode in Google Meet. (This isn’t native to Google Meet yet.)
tl,dr: ➡️ Link to the extension repo ⬅️.
Testing with an MVP 🧪
With the help of a QuerySelector directly from an open Google Meet tab, I was able to test the feasibility of this idea.
To grab the “Open PiP” button in the options menu and click it:
document
.evaluate(
"//span[contains(., 'picture-in-picture')]",
document,
null,
XPathResult.ANY_TYPE,
null
)
.iterateNext()
.click();
Test passed! ✅
However it seemed that the options menu had to be open first else the click event would not work.
So let’s grab the ‘more options’ menu before clicking our button.
Here are the XPaths for both the ‘more options’ and ‘picture-in-picture’ buttons:
const MORE_OPTIONS_XPATH =
"//i[normalize-space(@class) = 'google-material-icons' and contains(., 'more_vert')]";
const PICTURE_IN_PICTURE_XPATH = "//span[contains(., 'picture-in-picture')]";
I noticed that the ‘more options’ button is a bit slow to open the menu, as if the options were being fetched from the server each time it was clicked. Weird? 🤔
Anyway, I added a delay before clicking the ‘picture-in-picture’ button else it would not find it straight away.
Wrapping into a Chrome Extension 🧰
Chrome extensions are so underrated piece of software: imagine bulding a platform allowing your end users to interact with anything on the web in a way that suits them best.
Just the concept of injecting scripts into any page is so powerful but we know with great power comes great responsibility 🕸️, that’s why browser extensions are heavily regulated and it can be cumbersome to even start building one.
Hopefully I previously made a boilerplate to help me get started with the manifest and background scripts and this use case is not too complex so I can keep it simple.
With our recent findings, it’s time to wrap this into a Chrome extension to run on Google Meet pages and bind the keyboard shortcut. I decided to go with “ctrl + p” to trigger the Picture-in-Picture mode since it’s not already in use by Google Meet and Chrome already uses “alt + p” for its native Picture-in-Picture mode.
Finally let’s remember our test from earlier: we need to add that little delay to ensure the menu is fully opened before clicking the ‘picture-in-picture’ button.
//content-script.js
document.addEventListener("keydown", function (event) {
if (event.ctrlKey && event.key === "p") {
let moreOptions = document
.evaluate(MORE_OPTIONS_XPATH, document)
.iterateNext();
moreOptions.click();
setTimeout(function () {
document
.evaluate(PICTURE_IN_PICTURE_XPATH, document)
.iterateNext()
.click();
}, 250); // 250ms seemed to work fine
}
});
// manifest.json
{
"name": "Meet Picture-in-Picture shortcut",
"version": "1.0",
"manifest_version": 3,
"background": {
"service_worker": "sw.js"
},
"permissions": ["scripting", "webNavigation"],
"host_permissions": ["https://meet.google.com/*"],
"action": {}
}
// sw.js
chrome.webNavigation.onDOMContentLoaded.addListener(async ({ tabId, _ }) => {
chrome.scripting.executeScript({
target: { tabId },
files: ["content-script.js"]
});
});
The extension is now complete and can be installed in our browser to start using the shortcut.
Follow through the README if you want to try it out yourself!